Substructural type system
|
Read other articles:

Aanloop tot de Russische invasie van Oekraïne in 2022 Onderdeel van Russisch-Oekraïense Oorlog Kaart met aanduiding van Russische troepen in de Russisch-Oekraïense grensstreek (december 2021) Datum 3 maart 2021 – 24 februari 2022 Strijdende partijen Rusland Wit-Rusland Oekraïne Leiders en commandanten Vladimir Poetin Aleksandr Loekasjenko Volodymyr Zelensky Troepensterkte 900.000 actief personeel; 2.000.000 reservisten[1], 175.000[2] – 190.000[3...

Maltese Pioneers Historia Państwo Zjednoczone Królestwo Wielkiej Brytanii i Irlandii Sformowanie 1800 Rozformowanie 1801 Dowódcy Pierwszy Lieutenant Francesco Rivarola Działania zbrojne Wojny rewolucyjnej Francji kampania egipska Organizacja Rodzaj sił zbrojnych British Army Rodzaj wojsk wojska inżynieryjne Maltese Pioneers (pol. Maltański Korpus Saperów) – korpus saperów w składzie British Army, który istniał od 1800 do 1801. W grudniu 1800 sir Ralph Abercromby polecił po...

Avery Robert Dulles Cardeal da Santa Igreja Romana Presbítero da Companhia de Jesus Atividade eclesiástica Congregação Companhia de Jesus Ordenação e nomeação Ordenação presbiteral 16 de junho de 1956por Francis Joseph Cardeal Spellman Cardinalato Criação 21 de fevereiro de 2001 por Papa João Paulo II Ordem Cardeal-diácono Título Santíssimos Nomes de Jesus e Maria na Via Lata Brasão Lema Scio cui credidi Dados pessoais Nascimento Auburn24 de agosto de 1918 Morte New York12 de...

Nama ini menggunakan cara penamaan Spanyol: nama keluarga pertama atau paternalnya adalah Tafur dan nama keluarga kedua atau maternalnya adalah Náder. Gabriela TafurLahirGabriela Tafur Náder07 Juli 1995 (umur 28)Cali, ColombiaAlmamaterUniversitas Los AndesTinggi179 m (587 ft 3 in)Pemenang kontes kecantikanGelarSrta. Colombia 2018Warna rambutCoklatWarna mataCoklatKompetisiutamaMiss Colombia 2018(Pemenang)Miss Universe 2019(5 besar) Gabriela Tafur Náder (lahir 7 Juli...

1960 filmGoliath and the DragonDirected byVittorio CottafaviScreenplay by Marcello Baldi Duccio Tessari Mario Ferrari Nicolo Ferrari Fabio Carpi Ennio De Concini Franco Rossetti[2] Story by Marcello Baldi Nicolo Ferrari[2] Produced by Gianni Fuchs Achille Piazzi[2] Starring Mark Forest Broderick Crawford Gaby André Renato Terra CinematographyMario Montuori[2]Music byAlexandre Derevitsky[2]Productioncompanies Achille Piazzi Produzioni Cinematografica Pr...

Vowel sound represented by ⟨ɐ⟩ in IPA Near-open central vowelɐIPA Number324Audio sample source · helpEncodingEntity (decimal)ɐUnicode (hex)U+0250X-SAMPA6Braille Image IPA: Vowels Front Central Back Close i y ɨ ʉ ɯ u Near-close ɪ ʏ ʊ Close-mid e ø ɘ ɵ ɤ o Mid e̞ ø̞ ə ɤ̞ o̞ Open-mid ɛ œ ɜ ɞ ʌ ɔ Near-open æ ɐ Open a ɶ ä ɑ ɒ IPA help audio full chart template Legend: unrounded • rounded The near-open central vowel, or...

Not to be confused with Beaufort, South Carolina or Beaufort County, North Carolina. Town in North Carolina, United StatesBeaufort, North CarolinaTownDowntown Beaufort FlagSealLocation of Beaufort, North CarolinaCoordinates: 34°43′21″N 76°39′01″W / 34.72250°N 76.65028°W / 34.72250; -76.65028CountryUnited StatesStateNorth CarolinaCountyCarteretNamed forHenry Somerset, Duke of BeaufortArea[1] • Total7.84 sq mi (20.31 km2)...

European Network on Debt and DevelopmentAbbreviationEurodadFormation1990TypeCoalition of NGOsHeadquartersRue d’Edimbourg, 18 – 26,LocationBrusselsDirectorJean SaldanhaWebsitewww.eurodad.org Eurodad (European Network on Debt and Development) is a network of 53 non-governmental organisations and seven statutory allies from 29 European countries.[1] Eurodad and its members make up a network, this network researches and works on issues that are related to debt, development finance and...

2004 film by Alfonso Cuarón Harry Potter and the Prisoner of AzkabanTheatrical release posterDirected byAlfonso CuarónScreenplay bySteve KlovesBased onHarry Potter and the Prisoner of Azkabanby J. K. RowlingProduced by David Heyman Chris Columbus Mark Radcliffe Starring Daniel Radcliffe Rupert Grint Emma Watson Robbie Coltrane Michael Gambon Richard Griffiths Gary Oldman Alan Rickman Fiona Shaw Maggie Smith Timothy Spall David Thewlis Emma Thompson Julie Walters CinematographyMichael Seresi...

National sports governing body for basketball in Great Britain This article includes a list of references, related reading, or external links, but its sources remain unclear because it lacks inline citations. Please help to improve this article by introducing more precise citations. (May 2018) (Learn how and when to remove this template message) British Basketball FederationAbbreviationBBFPredecessorBritish & Irish Basketball FederationFormation2006TypeNational sports governing bodyLegal ...

Operation MerlynPart of the South African Border WarLocationNamibiaTsumebGrootfonteinWindhoekRunduOshakatiEenhanaOperation Merlyn (Namibia)ObjectivePrevent the incursion of PLAN (SWAPO) insurgents into South West Africa/Namibia in contravention to a ceasefire which came into effect on 1 April 1989.Date1–9 April 1989 vteSouth African Border War 1960s Blouwildebees 1970s Alcora Exercise Savannah Quifangondo Seiljag Bruilof Reindeer Cassinga Rekstok Saffraan 1980s Sceptic Klipklop Wishbone Bea...

Nazi coverup of the Holocaust that fooled the Red Cross During World War II, the Theresienstadt concentration camp was used by the Nazi SS (German: Schutzstaffel) as a model ghetto[1] for fooling Red Cross representatives about the ongoing Holocaust and the Nazi plan to murder all Jews. The Nazified German Red Cross visited the ghetto in 1943 and filed the only accurate report on the ghetto, describing overcrowding and undernourishment. In 1944, the ghetto was beautified in preparatio...

American journalist Larry BenskyBensky in 2005Born (1937-05-01) May 1, 1937 (age 86)OccupationJournalist Larry Bensky (born May 1, 1937) is a literary and political journalist with experience in both print and broadcast media, as well as a teacher and political activist. He is known for his work with Pacifica Radio station KPFA-FM in Berkeley, California, and for the nationally-broadcast hearings he anchored for the Pacifica network. A native of New York City, Bensky graduated from Stuyv...
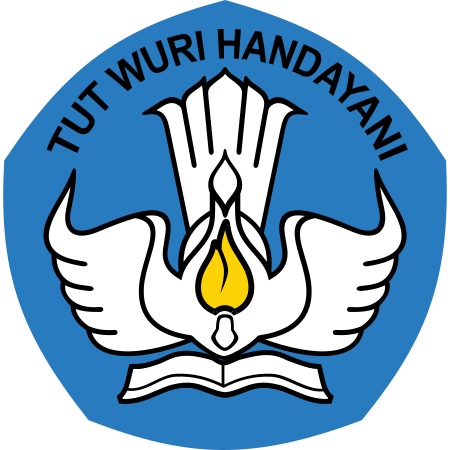
SDN 5 TilongkabilaSekolah Dasar Negeri 5 TilongkabilaInformasiAkreditasiBKepala SekolahJusra LadikuJumlah kelas11Rentang kelasI-VIStatusNegeriAlamatLokasiJln. HB. Yasin, Bone Bolango, Gorontalo, IndonesiaKoordinat0°33′00″N 123°07′49″E / 0.5500000°N 123.1302000°E / 0.5500000; 123.1302000Moto SD Negeri 5 Tilongkabila atau nama lengkapnya Sekolah Dasar Negeri 5 Tilongkabila adalah sebuah sekolah dasar umum yang terletak di jalan HB. Yasin, desa Mouto...

Italian painter (1569–1633) Lucio MassariThe Holy FamilyBorn22 January 1569BolognaDied3 November 1633(1633-11-03) (aged 64)NationalityItalianKnown forPaintingMovementMannerism and Baroque Lucio Massari (22 January 1569 – 3 November 1633) was an Italian painter of the School of Bologna. He can be described as painting during both Mannerist and early-Baroque periods. Life and work He was born in Bologna, where he initially apprenticed with an unknown painter by the name of Spi...

Maltz Opera HouseThe Maltz in 1988, as the State TheaterFormer namesAMC Classic State 3 Carmike State Cinemas 3 State Theater Maltz TheaterAddress206 North 2nd AvenueAlpena, MichiganUnited StatesCoordinates45°3′46.415″N 83°25′56.237″W / 45.06289306°N 83.43228806°W / 45.06289306; -83.43228806ConstructionBroke ground1876OpenedNovember 11, 1879; 144 years ago (1879-11-11)Rebuilt1925 The Maltz Opera House is a theater in Alpena, Michigan, name...

2015 American filmClingerDirected byMichael StevesWritten byGabi Chennisi DuncombeBubba FishMichael StevesStarringVincent MartellaJennifer LaporteJulia AksLisa WilcoxCinematographyGabi Chennisi DuncombeEdited byBubba FishMusic byMisha SegalProductioncompanyClinger The MovieRelease date January 12, 2015 (2015-01-12) (Slamdance Film Festival) Running time81 minutesCountryUnited StatesLanguageEnglish Clinger is a 2015 comedy horror film and the feature film directorial debut o...

Constituency of the State Duma of the Russian Federation Artyom single-member constituency Constituency of the Russian State DumaDeputyVladimir NovikovUnited RussiaFederal subjectPrimorsky KraiDistrictsArtyom, Bolshoy Kamen, Chernigovsky, Fokino, Khorolsky, Mikhaylovsky, Shkotovsky, Spassk-Dalny, Spassky, Vladivostok (Pervorechensky, Sovetsky)[1]Voters472,391 (2021)[2] The Artyom constituency (No.63) is a Russian legislative constituency in Primorsky Krai. The constituency was...

سانت هيلير الإحداثيات 48°00′47″N 96°12′51″W / 48.013055555556°N 96.214166666667°W / 48.013055555556; -96.214166666667 [1] تقسيم إداري البلد الولايات المتحدة[2] التقسيم الأعلى مقاطعة بينينغتون، مينيسوتا خصائص جغرافية المساحة 2.185584 كيلومتر مربع2.163853 كيلومتر مربع (1 أبريل ...

For the former school at Mamhead, see Mamhead House. Academy in Dawlish, Devon, EnglandDawlish CollegeAddressElm Grove RoadDawlish, Devon, EX70BYEnglandCoordinates50°35′03″N 3°27′46″W / 50.584187°N 3.462659°W / 50.584187; -3.462659InformationTypeAcademyLocal authorityDevon County CouncilTrustIvy Education TrustDepartment for Education URN147643 TablesOfstedReportsHead of SchoolSam BanksExecutive PrincipalPaul CornishGenderCoeducationalAge11 to 16Website...